After the first introduction of the Script TOP, the coming example will implement the Canny Edge Detector with OpenCV in TouchDesigner as a demonstration. TouchDesigner already includes its own Edge TOP for edge detection and visualisation.
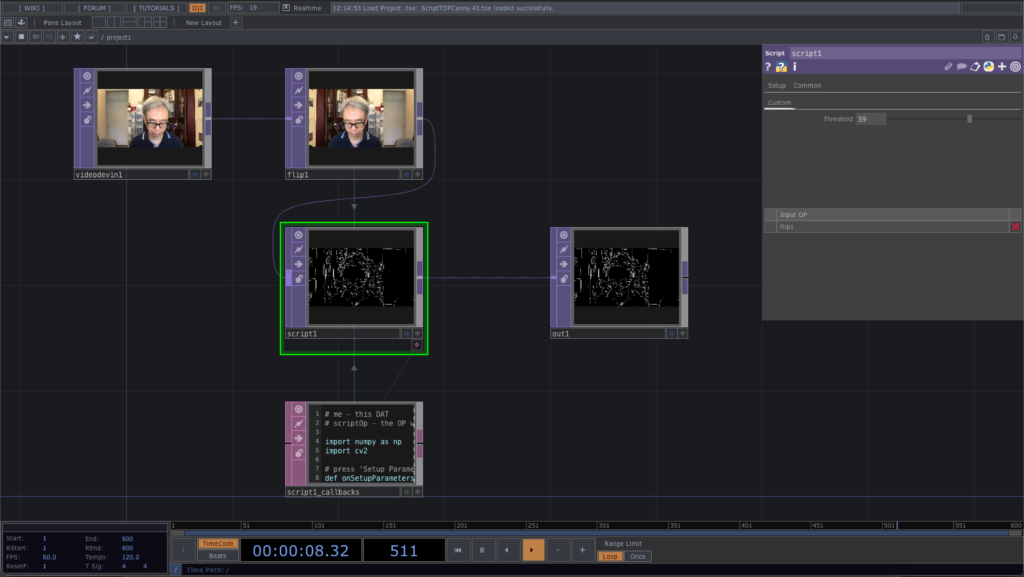
We also implement a slider parameter Threshold in the Script TOP to control the variation of edge detection.
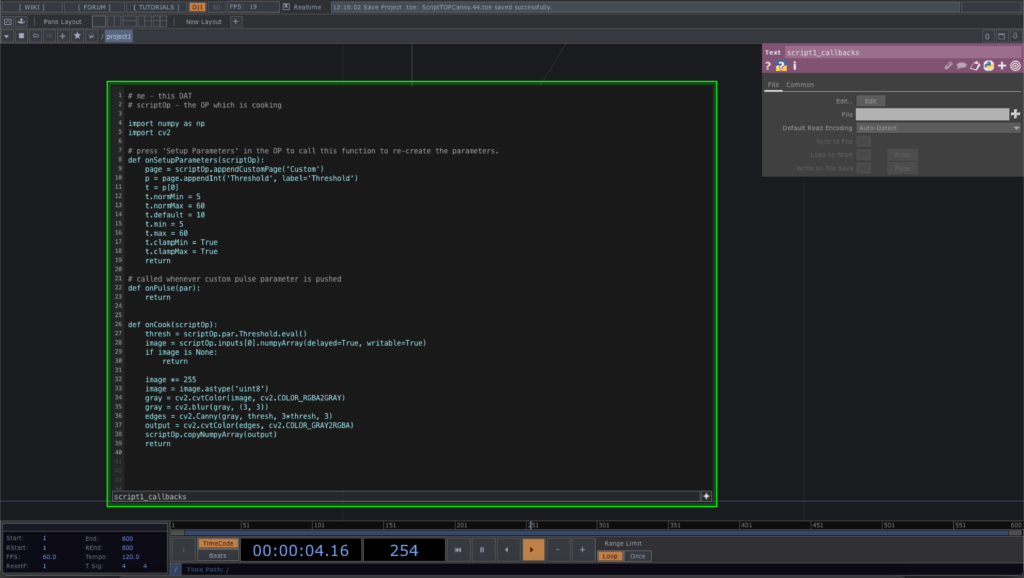
Here is the source code of the Script TOP. Note that we have made a lot of changes in the default function, onSetupParameters to include a custom parameter, Threshold as an integer slider. It will generate a value between 5 and 60, to be used in the onCook function as a threshold value for the Canny edge detection.
# me - this DAT # scriptOp - the OP which is cooking import numpy as np import cv2 # press 'Setup Parameters' in the OP to call this function to re-create the parameters. def onSetupParameters(scriptOp): page = scriptOp.appendCustomPage('Custom') p = page.appendInt('Threshold', label='Threshold') t = p[0] t.normMin = 5 t.normMax = 60 t.default = 10 t.min = 5 t.max = 60 t.clampMin = True t.clampMax = True return # called whenever custom pulse parameter is pushed def onPulse(par): return def onCook(scriptOp): thresh = scriptOp.par.Threshold.eval() image = scriptOp.inputs[0].numpyArray(delayed=True, writable=True) if image is None: return image *= 255 image = image.astype('uint8') gray = cv2.cvtColor(image, cv2.COLOR_RGBA2GRAY) gray = cv2.blur(gray, (3, 3)) edges = cv2.Canny(gray, thresh, 3*thresh, 3) output = cv2.cvtColor(edges, cv2.COLOR_GRAY2RGBA) scriptOp.copyNumpyArray(output) return
The first line in the onCook function is to retrieve the integer value from the parameter, Threshold. We also exit the function when there is not valid video image coming in. For the edge detection, we convert the RGBA image into grayscale and then perform a blur function. the cv2.Canny function returns the detected edges in a grayscale image, edges. Finally, we convert the edges into a regular RGBA image, output, for subsequent output as before.
The final TouchDesign project is available in this GitHub repository.