The major references for video capture in Cocoa are the QTKit Application Programming Guide, and the QTKit Application Tutorial. They have the basic examples to display the camera view in the Cocoa window.
The Simple QTKit Recorder Application is the starting point. I’ll further simplify it to remove the two buttons. Here is the layout sketch from the original sample.
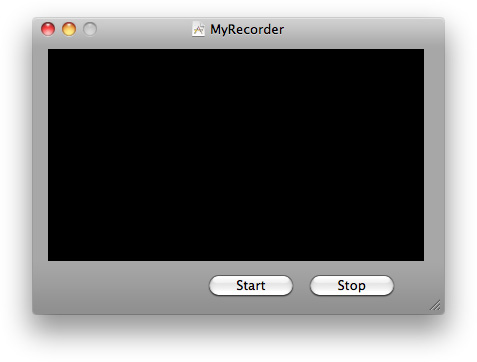
Here is the XCode 4.0 project folder.
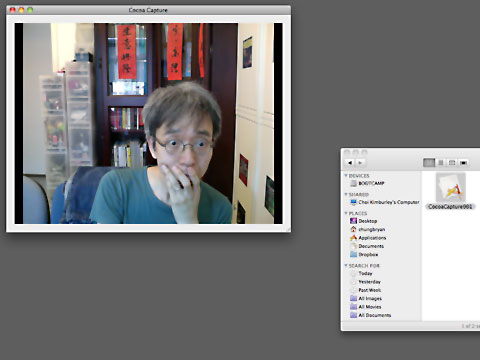
The header file
#import <Foundation/Foundation.h>
#import <QTKit/QTKit.h>
@interface MyRecorderController : NSObject {
@private
IBOutlet QTCaptureView *mCaptureView;
QTCaptureSession *mCaptureSession;
QTCaptureDeviceInput *mCaptureDeviceInput;
}
@end |
#import <Foundation/Foundation.h>
#import <QTKit/QTKit.h>
@interface MyRecorderController : NSObject {
@private
IBOutlet QTCaptureView *mCaptureView;
QTCaptureSession *mCaptureSession;
QTCaptureDeviceInput *mCaptureDeviceInput;
}
@end
The objective-c file
#import "MyRecorderController.h"
@implementation MyRecorderController
- (id)init
{
self = [super init];
if (self) {
// Initialization code here.
}
return self;
}
- (void)dealloc
{
[mCaptureSession release];
[mCaptureDeviceInput release];
[super dealloc];
}
- (void)awakeFromNib
{
mCaptureSession = [[QTCaptureSession alloc] init];
BOOL success = NO;
NSError *error;
QTCaptureDevice *device = [QTCaptureDevice defaultInputDeviceWithMediaType:QTMediaTypeVideo];
if (device) {
success = [device open:&error];
if (!success) {
NSLog(@"Device open error.");
}
mCaptureDeviceInput = [[QTCaptureDeviceInput alloc] initWithDevice:device];
success = [mCaptureSession addInput:mCaptureDeviceInput error:&error];
if (!success) {
NSLog(@"Add input device error.");
}
[mCaptureView setCaptureSession:mCaptureSession];
[mCaptureSession startRunning];
}
}
- (void)windowWillClose:(NSNotification *)notification
{
[mCaptureSession stopRunning];
[[mCaptureDeviceInput device] close];
}
@end |
#import "MyRecorderController.h"
@implementation MyRecorderController
- (id)init
{
self = [super init];
if (self) {
// Initialization code here.
}
return self;
}
- (void)dealloc
{
[mCaptureSession release];
[mCaptureDeviceInput release];
[super dealloc];
}
- (void)awakeFromNib
{
mCaptureSession = [[QTCaptureSession alloc] init];
BOOL success = NO;
NSError *error;
QTCaptureDevice *device = [QTCaptureDevice defaultInputDeviceWithMediaType:QTMediaTypeVideo];
if (device) {
success = [device open:&error];
if (!success) {
NSLog(@"Device open error.");
}
mCaptureDeviceInput = [[QTCaptureDeviceInput alloc] initWithDevice:device];
success = [mCaptureSession addInput:mCaptureDeviceInput error:&error];
if (!success) {
NSLog(@"Add input device error.");
}
[mCaptureView setCaptureSession:mCaptureSession];
[mCaptureSession startRunning];
}
}
- (void)windowWillClose:(NSNotification *)notification
{
[mCaptureSession stopRunning];
[[mCaptureDeviceInput device] close];
}
@end