Instead of using the Processing millis() function or the Java Timer class, we can also make use of the relatively new Instant and Duration classes in Java 8. Here is one simple example for demonstration.
The program uses 2 Instant variables: start, end. It computes the time duration between them using the Duration.between() function.
import java.time.Instant;
import java.time.Duration;
Instant start;
PFont font;
void setup() {
size(640, 480);
start = Instant.now();
frameRate(30);
font = loadFont("AmericanTypewriter-24.vlw");
textFont(font, 24);
}
void draw() {
background(0);
Instant end = Instant.now();
Duration elapsed = Duration.between(start, end);
long ns = elapsed.toNanos();
text(Long.toString(ns), 230, 230);
} |
import java.time.Instant;
import java.time.Duration;
Instant start;
PFont font;
void setup() {
size(640, 480);
start = Instant.now();
frameRate(30);
font = loadFont("AmericanTypewriter-24.vlw");
textFont(font, 24);
}
void draw() {
background(0);
Instant end = Instant.now();
Duration elapsed = Duration.between(start, end);
long ns = elapsed.toNanos();
text(Long.toString(ns), 230, 230);
}
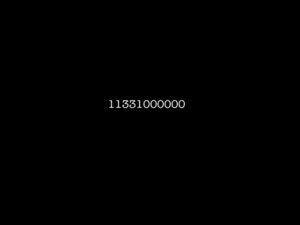